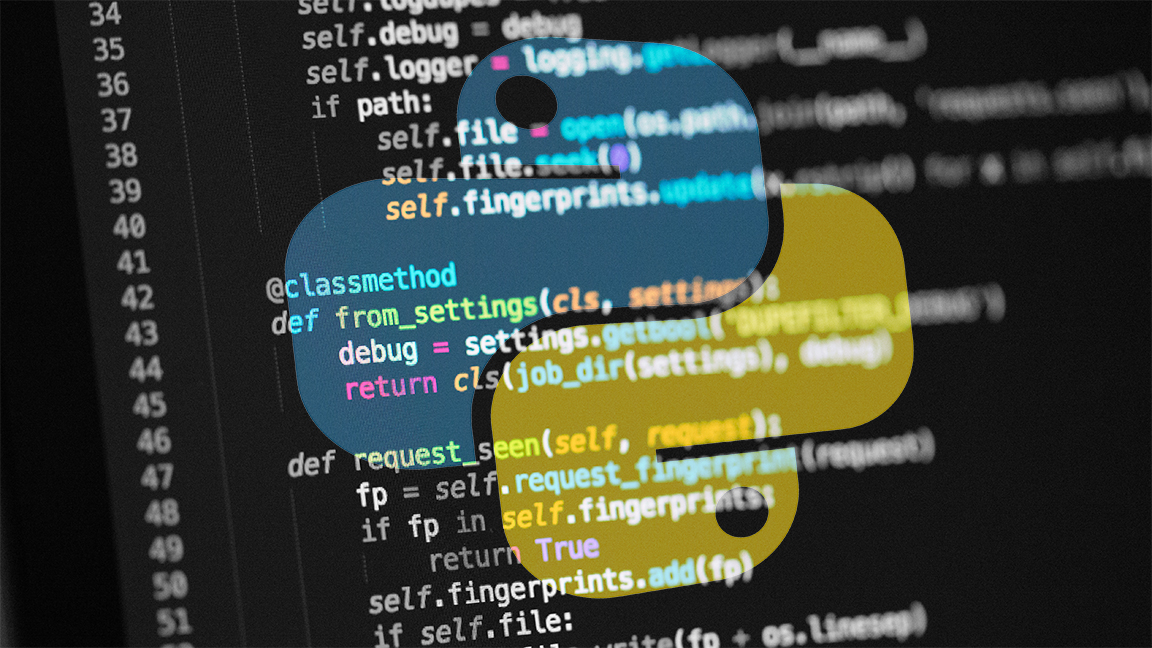
How to use financial market APIs in Python
API stands for application programming interface, a set of rules, protocols, and procedures that software developers can use to create new applications or integrate existing applications with other platforms. When you use an API to build an application, you get access to the other company’s database. There are several platforms that provide Financial market APIs, such as Alpha Vantage (AV), Twelve Data, and IEX Cloud. Working with the financial market APIs not only gives you access to historical data, but also you can use them to create your own trading or analyzing applications.
Here, I have explained briefly how to work with Alpha Vantage’s APIs. The procedure is kind of similar if using other platforms’ APIs, but you need to go through their documentation. To access AV’s API, first, you need to sign up on their website. For educational purposes, you can open a free account, however, if you plan to do serious work or develop an application, you might need to consider a premium account.
After subscribing, they will provide you a unique “Token”, which is like a passcode to be able to get the APIs. You can store the Token in a python constant.
AV_API_TOKEN = "3O3I5LC2JGXD6ZJ6" #the Token provided by the AV
Probably, a better practice is to put that variable in a python file (filename.py), place it in the same folder of your program, and call it (importing the module). In this way, you can share your programs, without being worried about sharing your Token. If you are using Jupiter Notebook or similar platforms, it is straightforward, however, if you are using Google Colab, you need to add extra lines of code to identify the file path.
Now, to call the AV’s APIs in python, we need to install their package:
#these lines of codes are just for the users of google colab
from google.colab import drive
drive.mount('/content/gdrive')
import sys
sys.path.insert(0,'/content/gdrive/MyDrive/')
import tokenkey #calling tokenkey.py module
from tokenkey import AV_API_TOKEN
Now, to use the AV’s APIs in python, we need to install their package. Please note that if you use Jupiter Notebook, the code is slightly different.
#Goggle Colab:
pip install alpha_vantage
#Joupiter Notebook:
#!pip install alpha_vantage
After installing the AV package, we need to import their libraries. There are two important libraries to get the time series data and technical indicators.
from alpha_vantage.timeseries import TimeSeries
from alpha_vantage.techindicators import TechIndicators
Before continuing with the AV’s APIs, let’s import some necessary libraries in python.
import pandas as pd
import numpy as np
pd.options.display.float_format = '{:,.5f}'.format #limited the float numbers to 5 digits after the decimal point
Time Series Stock Data APIs-Intraday
To get the stock data through the AV’s APIs, we need to indicate which token we are interested in and what is the ticker interval. The token in the stock market is a symbol that indicates the stocks of each company in the market. For example, the token of Apple company is “AAPL”. The ticker interval determines the frequency with which the APIs return the time-related prices. So, if we choose the “5min” ticker interval, the API returns the opening and closing prices in a 5 minutes interval, including the maximum/minimum of the price in that time interval, and also the whole volume of traded stocks.
symbol = 'AAPL'
ticker_interval = '5min' #the following values are supported: 1min, 5min, 15min, 30min, 60min
ts = TimeSeries(AV_API_TOKEN, output_format= 'pandas')
data, meta_data = ts.get_intraday(symbol, interval=ticker_interval, outputsize='full')
data.head()
1. open | 2. high | 3. low | 4. close | 5. volume | |
---|---|---|---|---|---|
date | |||||
2022-10-05 20:00:00 | 146.97000 | 147.40000 | 146.91000 | 147.31000 | 21,831.00000 |
2022-10-05 19:55:00 | 146.80000 | 146.98000 | 146.73000 | 146.97000 | 9,070.00000 |
2022-10-05 19:50:00 | 146.69000 | 146.87000 | 146.69000 | 146.82000 | 7,817.00000 |
2022-10-05 19:45:00 | 146.50000 | 146.64000 | 146.50000 | 146.62000 | 4,018.00000 |
2022-10-05 19:40:00 | 146.44000 | 146.50000 | 146.44000 | 146.50000 | 3,338.00000 |
By default, the AV returns the adjusted prices. If you are interested in the raw prices, you need to use this option: adjusted=false
data, meta_data = ts.get_intraday(symbol, interval=ticker_interval, adjusted=false outputsize='full')
Now, let’s change the column names, sparse the time series index, and remove after-hour market prices from our data.
stock_data = data.copy()
columns = ['Open', 'High', 'Low', 'Close', 'Volume']
stock_data.columns = columns
stock_data['TradeDate'] = stock_data.index.date #adding seperate time and date columns
stock_data['Time'] = stock_data.index.time
stock_data = stock_data.between_time('09:30:00', '16:00:00').copy() #USA stock market is operating from 9:30 am ET to 4:00 pm ET
stock_data.sort_index(inplace=True)
stock_data.head()
Open | High | Low | Close | Volume | TradeDate | Time | |
---|---|---|---|---|---|---|---|
date | |||||||
2022-09-07 09:30:00 | 155.08000 | 155.11000 | 154.53000 | 154.81000 | 93,285.00000 | 2022-09-07 | 09:30:00 |
2022-09-07 09:35:00 | 154.82500 | 155.68000 | 154.75000 | 155.26000 | 3,134,859.00000 | 2022-09-07 | 09:35:00 |
2022-09-07 09:40:00 | 155.26000 | 155.75000 | 155.10000 | 155.60000 | 1,805,040.00000 | 2022-09-07 | 09:40:00 |
2022-09-07 09:45:00 | 155.61000 | 155.91620 | 155.14000 | 155.44000 | 1,716,995.00000 | 2022-09-07 | 09:45:00 |
2022-09-07 09:50:00 | 155.43000 | 155.79000 | 155.40010 | 155.65000 | 1,224,542.00000 | 2022-09-07 | 09:50:00 |
Extended intraday time series data
As can be seen, in this way, we can get the time series data of Apple company’s stocks for the last month. If we are interested to get more historic data, it is available by Intraday-Extended API, which can return the monthly time series data up to two years ago. For some reason, this function does not work properly through the AV API. However, we can get access to the APIs directly through their URLs.
symbol = 'AAPL'
ticker_interval = '60min'
ticker_slice = 'year2month1' #from AV documentation: intraday data is evenly divided into 24 slices, 'year1month1', 'year1month2', ..., 'year2month12'
#each slice is a 30-day window, with 'year1month1' being the most recent and year2month12 being the farthest from today
api_url = f'https://www.alphavantage.co/query?function=TIME_SERIES_INTRADAY_EXTENDED&symbol={symbol}&interval={ticker_interval}&slice={ticker_slice}&apikey={AV_API_TOKEN}'
data_ext = pd.read_csv(api_url)
stock_data_ext = data_ext.set_index('time')
stock_data_ext.index = pd.to_datetime(stock_data_ext.index)
columns = ['Open', 'High', 'Low', 'Close', 'Volume']
stock_data_ext.columns = columns #changing the columns names
stock_data_ext['TradeDate'] = stock_data_ext.index.date #adding seperate time and date columns
stock_data_ext['time'] = stock_data_ext.index.time
stock_data_ext = stock_data_ext.between_time('09:30:00', '16:00:00').copy() #USA stock market is operating from 9:30 am ET to 4:00 pm ET
stock_data_ext.sort_index(inplace=True)
stock_data_ext.head()
Open | High | Low | Close | Volume | TradeDate | time | |
---|---|---|---|---|---|---|---|
time | |||||||
2021-09-13 10:00:00 | 149.66257 | 150.57745 | 148.86702 | 149.03607 | 19171561 | 2021-09-13 | 10:00:00 |
2021-09-13 11:00:00 | 149.02613 | 149.40899 | 148.22064 | 149.22402 | 16835779 | 2021-09-13 | 11:00:00 |
2021-09-13 12:00:00 | 149.22502 | 149.56810 | 148.61841 | 148.65819 | 10149777 | 2021-09-13 | 12:00:00 |
2021-09-13 13:00:00 | 148.65819 | 149.27464 | 148.55874 | 149.19518 | 8423209 | 2021-09-13 | 13:00:00 |
2021-09-13 14:00:00 | 149.19021 | 149.28468 | 147.99192 | 148.13114 | 8761173 | 2021-09-13 | 14:00:00 |
Daily and monthly time series data
Similar to the intraday time series stock market data, you can get daily and monthly adjusted data through the APIs of Alpha Vantage.
Update: These features are not available for the free account anymore.
symbol = 'AAPL'
ts = TimeSeries(AV_API_TOKEN, output_format= 'pandas')
daily_data_adj, meta_daily_adj = ts.get_daily_adjusted(symbol, outputsize= 'full')
monthly_data_adj, meta_monthly_adj = ts.get_monthly_adjusted(symbol, outputsize= 'full')
Technical indicators’ time series data
Furthermore, you can get the technical indicators’ time series data, directly through the AV’s APIs. The below code is for getting the Simple moving average for a “time-period” of 15 tickers on the “close” prices. value of the ticker is determined by the ticker interval.
Update: this feature is not available for the free accounts anymore.
symbol = 'AAPL'
ticker_interval = '5min' #the following values are supported: '1min', '5min', '15min', '30min', '60min', 'daily', 'weekly', 'monthly'
ti = TechIndicators(AV_API_TOKEN, output_format='pandas')
sma_data, meta = ti.get_sma(symbol, interval=ticker_interval, time_period=15, series_type='close')